Data Layer
The data layer plays a crucial role in any application by managing the core data and the business logic that governs how this data is handled.It operates separately from the UI layer, focusing on the real-world rules and requirements that define how information is created, stored, and modified within the app.While the UI layer handles how data is presented to the user, the data layer manages the actual state of the application. It ensures data integrity and handles interactions with databases, APIs, or other external sources.Business logic, a key component of the data layer, encapsulates the rules and processes that give value to the app. These are the guidelines that determine how data is manipulated, such as validating user input, calculating results, or ensuring correct data formatting before it’s presented or stored.
Separating the data layer from the UI has several advantages:
- It allows the data to be reused across multiple screens and components.
- It enables different parts of the app to share information more effectively.
- Business logic can be easily tested outside of the UI, improving the ability to write unit tests and ensure code quality without involving the interface.
Data Layer Architecture
The data layer in an application is structured around repositories, which serve as intermediaries between the app’s business logic and the various data sources.Each repository is designed to manage a specific type of data, centralizing the logic for how that data is retrieved, stored, or manipulated.A repository can contain multiple data sources or none at all, depending on the complexity of the app. For example, a repository might interact with a remote API, a local database, or even in-memory data, and consolidate all these sources into a unified interface for the app to consume.To maintain clarity and organization, you should create a dedicated repository class for each distinct type of data in your app.By structuring the data layer this way, each repository encapsulates its own logic and data handling, making the app more modular and easier to maintain. It also allows different repositories to work independently or in tandem, promoting clean code architecture and separation of concerns across the app.
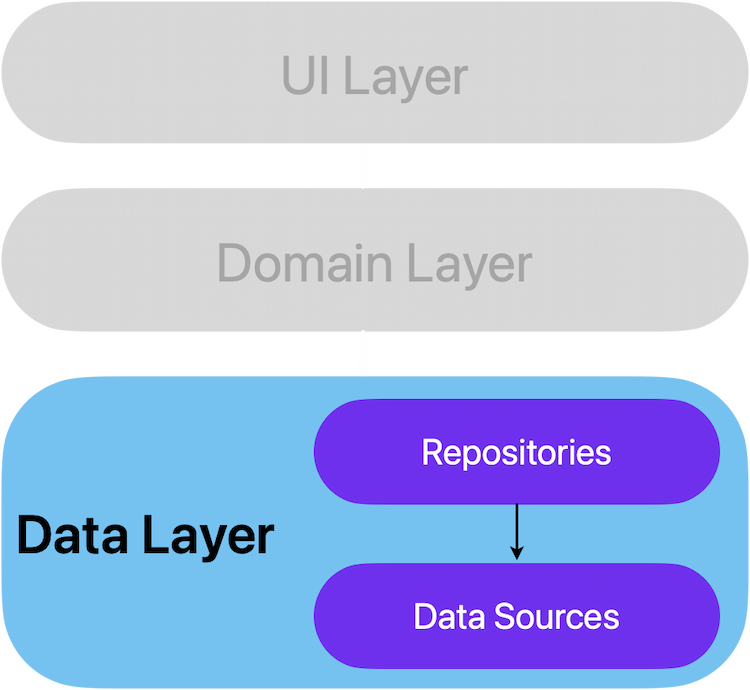
Data Sources
Each data source class should focus exclusively on handling a single type of data source, whether it’s interacting with files, network APIs, or a local database.These classes serve as the bridge between your app and the system, executing specific data operations such as reading, writing, or fetching data.To maintain proper separation of concerns, other layers of the application should never access data sources directly. Instead, the entry points to the data layer are always through the repository classes.
This ensures that repositories manage the complexity of interacting with multiple data sources while providing a clean, unified interface for the rest of the app.For example, a ProductsRepository might interact with both a remote API to fetch product data and a local database to cache it, but the UI layer or business logic should not need to know how or where this data is stored or retrieved. They interact solely with the repository.
Repositories
In a well-structured Flutter app, repository classes play a critical role in managing data operations and acting as intermediaries between the app and its data sources. Each repository handles a specific type of data, such as products or orders, and ensures smooth communication between the app and various sources like APIs, databases, or local files.Repository classes provide several key benefits:
- Exposing data: They serve as the primary point of access for data, making it available to the rest of the app.
- Resolving conflicts: When working with multiple data sources (e.g., remote and local), repositories manage conflicts and prioritize which source to use.
- Centralizing changes: Repositories ensure that any data modification is consistently applied across all data sources.
- Containing business logic: Repositories manage the rules and processes for working with data, such as how it should be retrieved, updated, or saved, keeping this logic organized and separate from other parts of the app.
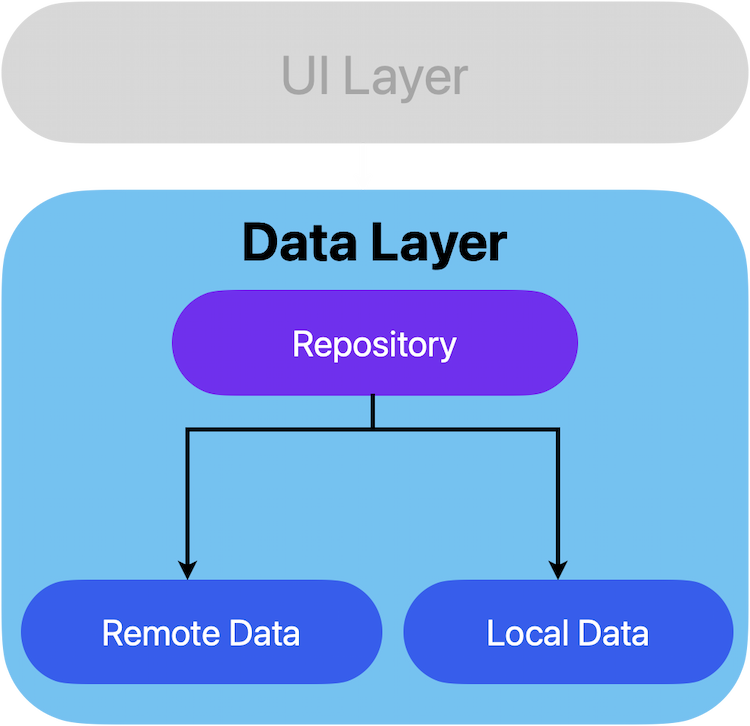