Data Sources (Remote)
In modern applications, integrating with remote servers via APIs is crucial for functionalities such as fetching data, submitting forms, and synchronizing information.In Flutter, the Retrofit package simplifies this process by providing a declarative approach to make HTTP requests, ensuring your code is clean, type-safe, and easy to maintain.
Below, we will walk through the setup of a remote data source using Retrofit specifically tailored for a Product model.
Retrofit: Remote Data Source
Retrofit is a type-safe HTTP client for Dart, which makes it easier to connect with web services.It abstracts the complexity of making network requests and handling responses, allowing developers to focus on the core functionality of their applications.
Retrofit uses annotations to define API endpoints, which automatically generate the necessary code for making HTTP calls. This declarative approach enhances readability and maintainability, as developers can clearly see how their application interacts with external services.
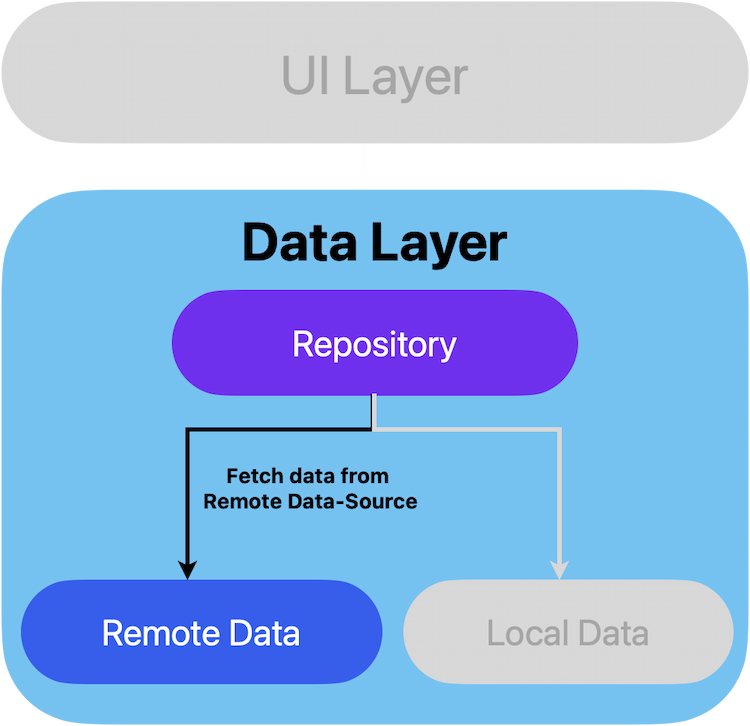
Define Product Remote Data-Source
This is a simple implementation of a REST client using Retrofit in Dart. The annotations like @GET, @PUT, @POST, and @DELETE define the API endpoints.Dio is used under the hood by Retrofit for making the HTTP requests.
import 'package:dio/dio.dart';
import 'package:retrofit/retrofit.dart';
import 'product.dart'; // your model
part 'products_remote_data_source.g.dart';
@RestApi()
abstract class ProductsRemoteDataSource {
factory ProductsRemoteDataSource(Dio dio) = _ProductsRemoteDataSource;
@POST('/products')
Future<BaseResponse<Product>> create({
@Body() required Map<String, dynamic> data,
@CancelRequest() CancelToken? cancelToken,
});
@PUT('/products/{id}')
Future<BaseResponse<Product>> update({
@Path('id') required int? id,
@Body() required Map<String, dynamic> data,
@CancelRequest() CancelToken? cancelToken,
});
@GET('/products')
Future<BaseResponse<ListResponse<Product>>> findAll({
@Query('page') required int page,
@Query('per_page') required int perPage,
@CancelRequest() CancelToken? cancelToken,
});
@GET('/products/{id}')
Future<BaseResponse<Product>> findOne({
@Path('id') required int? id,
@CancelRequest() CancelToken? cancelToken,
});
@DELETE('/products/{id}')
Future<BaseResponse<dynamic>> delete({
@Path('id') required int? id,
@CancelRequest() CancelToken? cancelToken,
});
}
dart run build_runner build --delete-conflicting-outputs